Loops are used to perform some action or block of code a certain amount of times. Loops simplify code by reducing the amount of repitition which makes it easier to read and understand. There are three main types of loops; the for loop, the while loop, and the do/while loop.
For Loop
This is best used when you know exactly how many times you need to execute a loop.
Each loop has conditions that tell the code where to start (initialize), how often it should be repeated (evaluate), and what it should count by (increment) each time.
This is the basic layout:
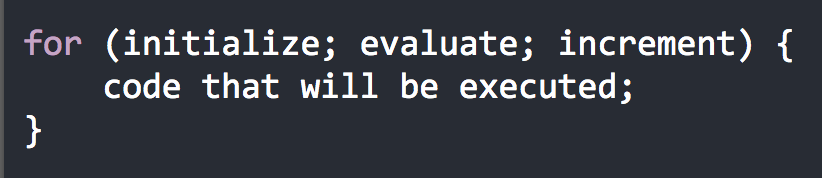
Example
Say you needed to output a list of years from a certain time period.
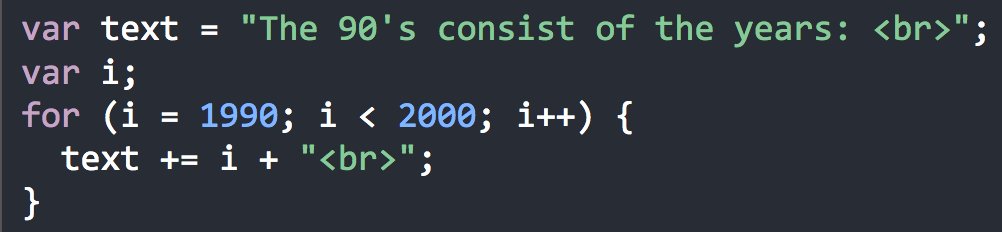
i=1990 - starts the year count at 1990.
i < 2000 - everytime the loop is run, it will check to see that each loop is less than 2000.
i++ - will add 1 to the year each time the loop finishes.
The output would be:
The 90's consist of the years:
1990
1991
1992
1993
1994
1995
1996
1997
1998
1999
While Loop
The While loop will execute a block of code, as long as the condition is true. The condition is tested before executing the block of code.
This is the basic layout:
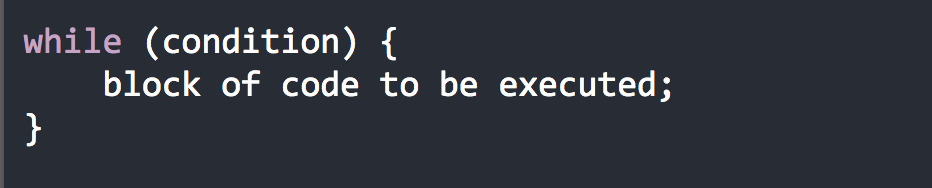
Example
Using the same example, we will list the dates of a certain time period.
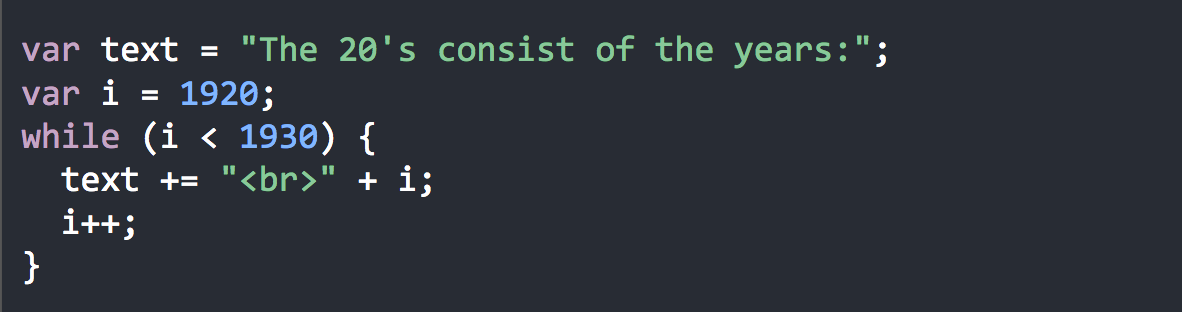
This time, the loop will first test to see if the condition "i < 30" is true. If it is true,
the block of code below will output the value of i, then increase the value of i by 1.
The output would be:
The 20's consist of the years:
1920
1921
1922
1923
1924
1925
1926
1927
1928
1929
Do/While Loop
The Do/While Loop is similar to the While Loop, however it will run the block of code once before it checks to see if the condition is true.
This is the basic format:
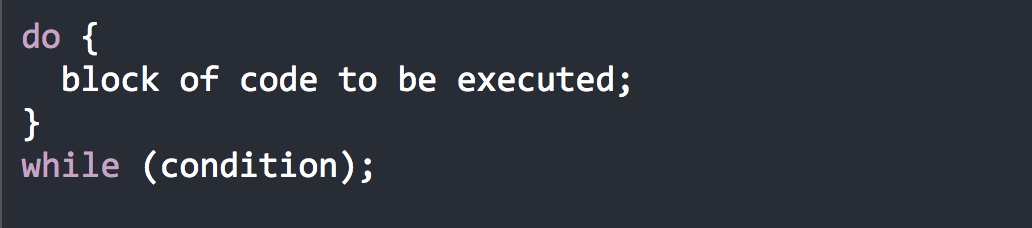
Example
We will stick with the time period example one last time.
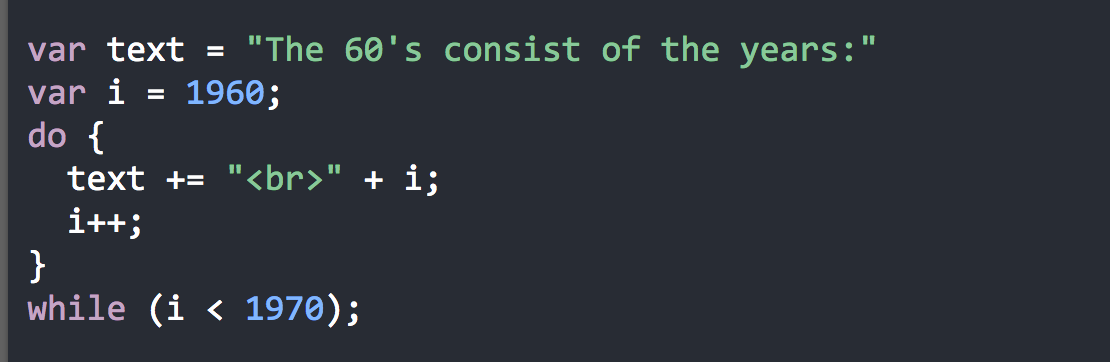
In this example, the block of code inside of do{} will be executed first. It will output the value
of i, then increase the valy of i by 1. Then it will check if the while() condition is true. If it
is not true, the code will stop.
The output would be:
The 60's consist of the years:
1960
1961
1962
1963
1964
1965
1966
1967
1968
1969