What is DOM?
DOM stands for Document Object Model. After the HTML page is loaded, the browser will create something called a Document Object Model of the page. This is what an example HTML DOM Tree of Objects looks like:
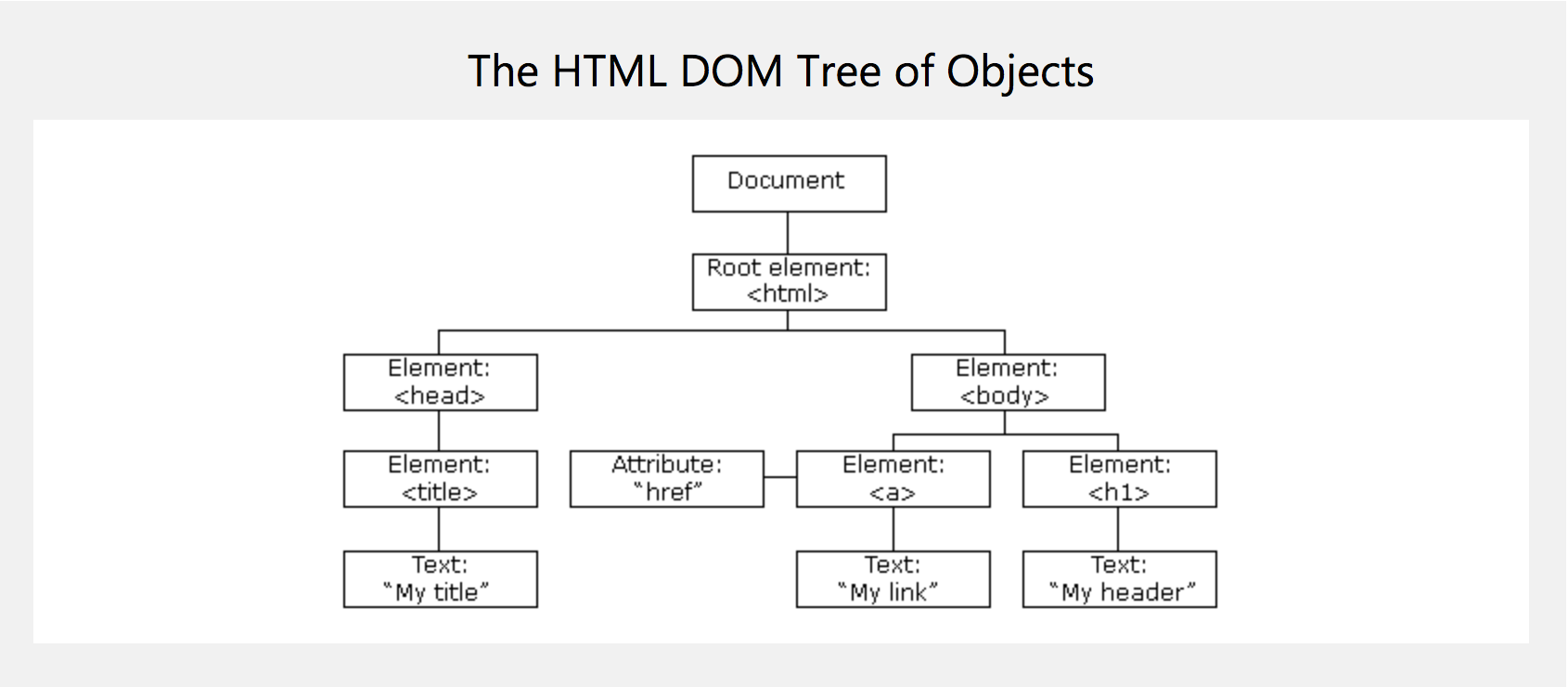
Within this model, it shows the document as the head element, then everything within html is the root element. This is further divided into the head element and the body element. Then each element inside of these tags is broken down.
By creating this object model, you can access any individual object using JavaScript to create, read, update, or delete elements and attributes within the HTML file. This becomes extremely useful when you want to make a static HTML page more dynamic.
I will explore some of the things you can do to manipulate HTML using JavaScript in the next few sections. Topic 7 shows how to use JavaScript to manipulate CSS class properties.